JavaScript is a high-level programming language that enjoys widespread adoption among developers globally. It has a global usage share of over 98.2% among web pages. Although renowned for its ease of use as an untyped language, novice programmers may encounter difficulties when implementing real-world projects.
If a company seeks to hire Javascript developers, they typically evaluate the candidates’ proficiency by posing these problems during the interview.
In this post, we shall delve into 8 common errors encountered by JavaScript programmers and ways to circumvent them.
JavaScript is a high-level programming language that enjoys widespread adoption among developers globally. It has a global usage share of over 98.2% among web pages. Although renowned for its ease of use as an untyped language, novice programmers may encounter difficulties when implementing real-world projects.
If a company seeks to hire Javascript developers, they typically evaluate the candidates’ proficiency by posing these problems during the interview.
In this post, we shall delve into 8 common errors encountered by JavaScript programmers and ways to circumvent them.
8 Common Mistakes JavaScript Developers Should Avoid
JavaScript is a versatile language that allows developers to build interactive and dynamic web pages that can respond to user input in real time. However, even experienced JavaScript developers can make mistakes that cause bugs, slow the application, or compromise security.
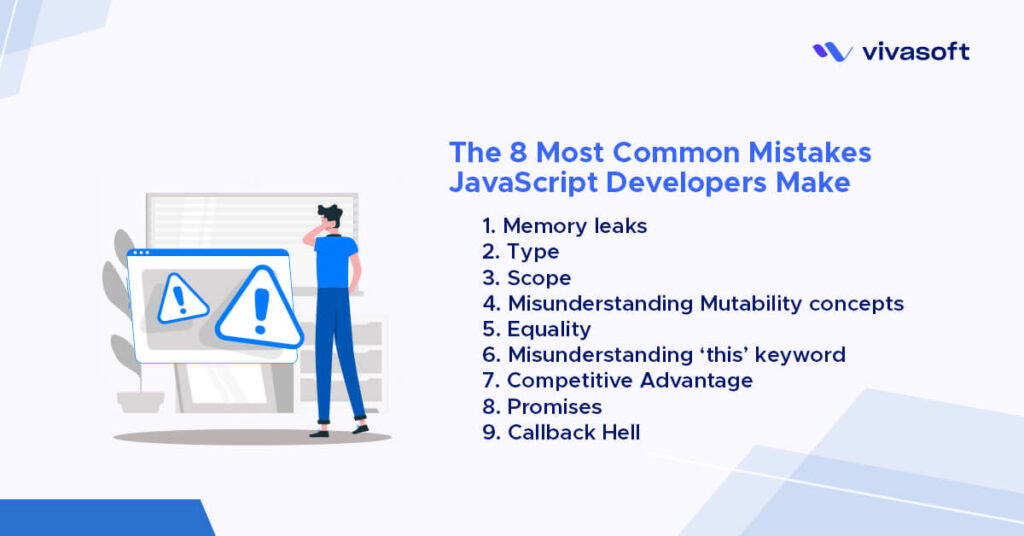
1. Memory leaks
A program may encounter memory leaks if it fails to deallocate memory that is no longer required, leading to a gradual increase in memory usage until the program crashes. This is a frequent error committed by JavaScript programmers who need to gain knowledge of JavaScript’s memory management and garbage collection principles.
Consider the following example code:
function createArray() {
var arr = new Array(1000000);
return arr;
}
var myArray = createArray();
function createArray() {
var arr = new Array(1000000);
return arr;
}
var myArray = createArray();
// Release memory
myArray = null;
2. Type
Javascript is not a strongly-typed language, which means that variables can hold different types of data at different times. However, this flexibility can lead to problems if you are a former C/C++ developer and not familiar with the type concept of Javascript.
Consider the following example:
var x = "10";
var y = 5;
var z = x + y;
var x = "10";
var y = 5;
var z = parseInt(x) + y;
3. Scope
The term “scope” refers to the portion of a program where a variable is reachable. In Javascript, variables declared inside a function have a function-level scope and are not accessible outside of it.
Consider the following example:
function myFunction() {
var x = 1;
}
myFunction();
console.log(x);
var x;
function myFunction() {
x = 1;
}
myFunction();
console.log(x);
4. Misunderstanding Mutability Concepts
In JavaScript, some data types such as strings and numbers are immutable, meaning that their value cannot be changed once they are created. On the other hand, objects and arrays are mutable, which means that their contents can be modified.
Consider the following example:
var x = 5;
var y = x;
y = 10;
console.log(x); // Output: 5
var x = [1, 2, 3];
var y = x;
y.push(4);
console.log(x); // Output: [1, 2, 3, 4]
const myObj = { foo: 'bar' };
myObj.foo = 'baz';
console.log(myObj); // Output: { foo: 'baz' }
Similarly, if you declare a const array, you can still modify its elements:
const myArr = [1, 2, 3];
myArr.push(4);
console.log(myArr); // Output: [1, 2, 3, 4]
5. Equality
console.log(5 == "5"); // Output: true
6. Misunderstanding ‘this’ keyword
var obj = {
x: 10,
getX: function() {
return this.x;
}
};
console.log(obj.getX()); // Output: 10
var getX = obj.getX;
console.log(getX()); // Output: undefined
7. Promises
Asynchronous programming is a fundamental component of JavaScript, and Promises are a popular method to manage asynchronous operations. A Promise represents a value that is currently unavailable but will become available in the future, either with a resolved value or a rejection reason.
There are three promise states:
- Pending: The initial state; neither the Promise is fulfilled nor is it rejected.
- Fulfillment: The process was completed, and the Promise’s value was resolved.
- Rejection: Because of the operation’s failure, the Promise is being rejected.
Here’s an example of a Promise that simulates a network request:
const getData = () => {
return new Promise((resolve, reject) => {
setTimeout(() => {
resolve("Data received successfully!");
}, 2000);
});
};
getData()
.then((data) => console.log(data))
.catch((error) => console.log(error));
8. Callback Hell
The term “callback hell” refers to a scenario in which there are numerous nested callbacks that render the code challenging to comprehend and sustain. This is a frequent error committed by Javascript programmers, particularly those who are inexperienced with asynchronous programming.
Here’s an example of what callback hell might look like-
getUser(userId, (user) => {
getProfile(user.profileId, (profile) => {
getPosts(profile.posts, (posts) => {
posts.forEach((post) => {
getComments(post.comments, (comments) => {
console.log(comments);
});
});
});
});
});
In this example, we have four levels of nested callbacks, resulting in code that is challenging to comprehend.
To reduce callback hell, one may utilize Promises, async/await, or libraries such as Async.js or Bluebird to achieve code that is more legible and sustainable.
Way out of these mistakes
If you aspire to become a Javascript developer, it is highly unlikely that you will not encounter any of these challenges during your early career as a developer. By carefully studying the Javascript documentation, such mistakes can be significantly reduced.
Fortunately, Javascript’s documentation is very thorough and well-crafted. By thoroughly studying and understanding it, you can circumvent numerous prevalent errors. Moreover, there exist plenty of online resources, tutorials, and JavaScript bootcamp that can furnish supplementary guidance and assistance as you acquire knowledge and advance as a Javascript programmer.
To minimize errors, it is recommended to practice good coding practices, such as producing tidy, structured, and sustainable code. Implementing early error detection can facilitate prompt issue identification and resolution.
Working with more experienced developers or participating in online communities and forums can also be valuable for getting feedback, advice, and support. Don’t be afraid to ask questions and seek out help when needed, as this can be an effective way to learn and avoid mistakes in the future.
Recommendation
If you are an employer who is looking for Javascript developers who hardly fall for such naive mistakes, our suggestion would be Vivasoft. Vivasoft has a team of seasoned Javascript developers for hire at your disposal for team augmentation.
To hire a dedicated developers team, contact us.
Final Thoughts
Dealing with Javascript errors can be quite a hassle and can take up a lot of your time. However, it’s important to note that these mistakes can actually serve as valuable learning opportunities that can aid in your growth and development as a skilled developer.
As a developer, it’s crucial to acknowledge that mistakes are inevitable. However, taking the time to learn from them and adopting good coding habits can make all the difference in building robust, reliable, and effective Javascript applications. By doing so, you can avoid many common issues that may arise during the development process.